BASIC SHAPES
// rect (position X, position Y, width, height);
rect (0,0,20,20);
// ellipse (position X, position Y, width, height);
ellipse (50,50,20,20);
// line (position X first point, position Y first point, position X second point, position Y second point);
line (10,10,90,90);
// point (position X, position Y);
point (70,70);
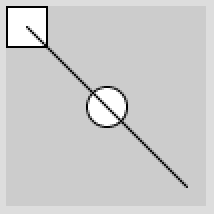
COLOR
// color fill shape gray
fill(125);
rect (10,10,40,40);
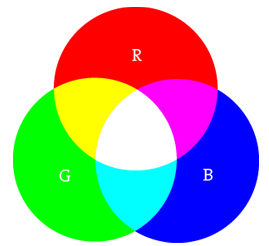
// color fill shape (Red,Green,Blue);
fill(0,255,0);
// color stroke shape (Red,Green,Blue);
stroke(255,0,0);
// Weight of stroke (pixel)
strokeWeight(20);
rect (10,10,40,40);
Use color selector to select a specific color
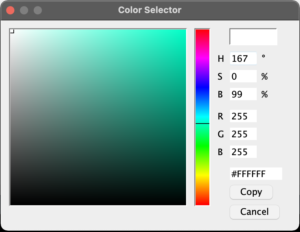
Custom color mode
You can chose the range of each value of the color at the beginning of your code.
//Red values from 0 to 100, green from 0 to 500, blue from 0 to 10, and alpha from 0 to 255.
colorMode(RGB,100,500,10,255);
Other color mode
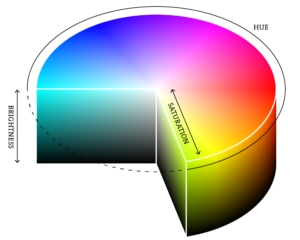
Hue—The color type, ranges from 0 to 255 by default.
Saturation—The vibrancy of the color, 0 to 255 by default.
Brightness—The, well, brightness of the color, 0 to 255 by default.
INTERACTION
To put interaction into your code you need to use two function “void setup” and “void draw”
//function that execute once at the begin of the code to setup basic element
void setup() {
size(100, 100);
background (255);
}
//function that execute the code inside in loop
void draw() {
//instead of using static value for position of the ellipse you can use the position of your mouse to define it (variables)
ellipse(mouseX, mouseY, 33, 33);
}
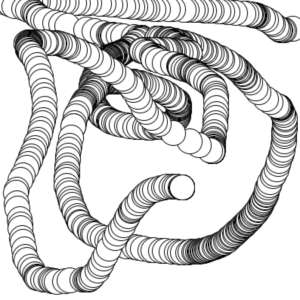
CONDITION
If you want to increase interaction in your code, you can start to add question into. Put some conditions and one way is to use “if” and “else”
void setup() {
size(400, 400);
background (255);
}
void draw() {
ellipse(mouseX, mouseY, 33, 33);
// If i pressed on my mouse my background will be fill in white
if (mousePressed) {
background (255);
}
}
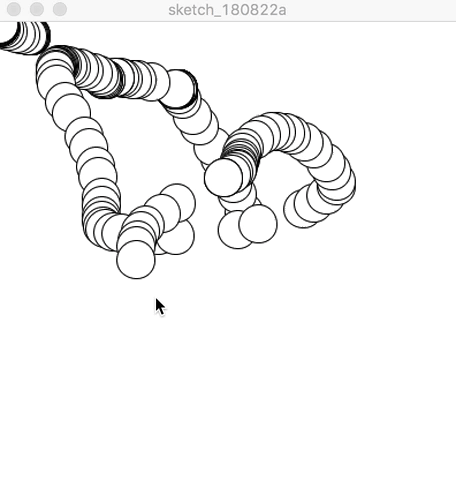
void setup() {
size(400, 400);
background (255);
}
void draw() {
if (mousePressed) {
fill(242,34,208);
}
else {
noStroke();
fill(142,255,15);
}
ellipse(mouseX, mouseY, 33, 33);
}
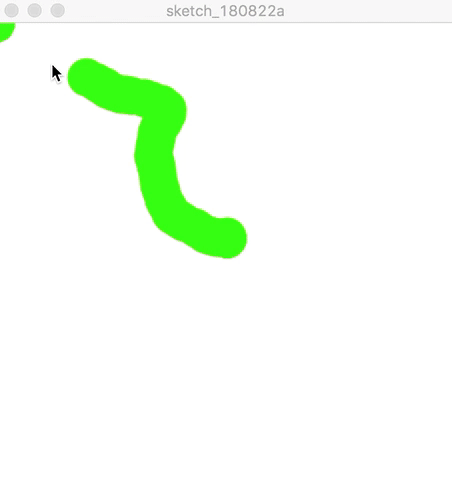
IMAGE
//Create object img
PImage img;
//boolean value to stop loop
boolean stop =true;
void setup() {
size(600, 600);
background(0);
// Images must be in the "data" directory to load correctly
img = loadImage("fish.png");
}
void draw() {
if (stop == true) {
// loop to do this action 10 times
for (int i=0; i<10; i++) {
image(img, random(width-240), random(height-112), 240, 112);
}
// switch the value state
stop = !stop;
}
}
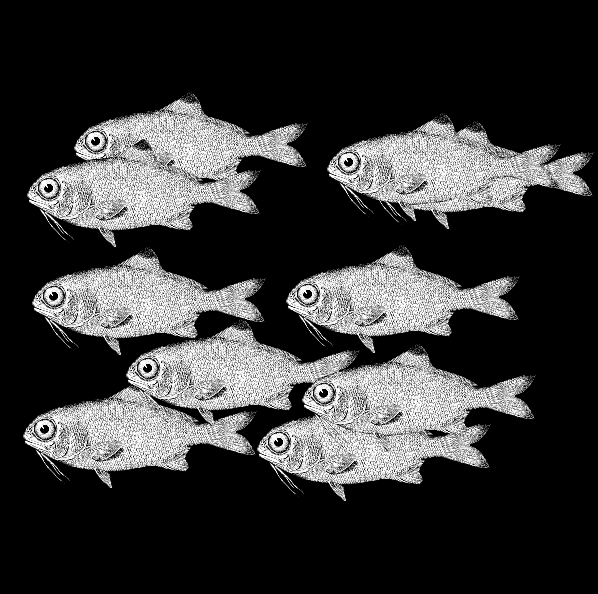
CAMERA
import processing.video.*;
Capture camera;
void setup() {
size(640, 480);
background(0);
String[] devices = Capture.list();
println(devices);
camera = new Capture(this, 640, 480, devices[0]);
camera.start();
}
void draw() {
if (camera.available()) {
camera.read();
image(camera, 0, 0);
filter(INVERT);
}
}
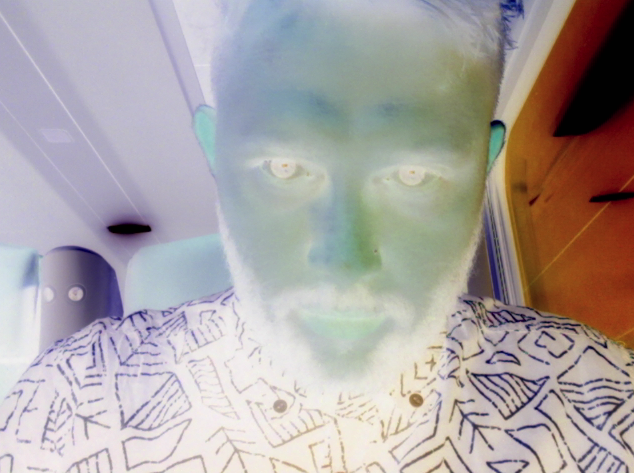
MICROPHONE
import processing.sound.*;
AudioIn input;
Amplitude loudness;
void setup() {
size(600, 600);
background(0);
input = new AudioIn(this, 0);
input.start();
loudness = new Amplitude(this);
loudness.input(input);
}
void draw() {
float volume = loudness.analyze();
int size = int(map(volume, 0, 0.5, 1, 600));
background(0);
noStroke();
fill(255, 0, 150);
ellipse(width/2, height/2, size, size);
println(inputLevel);
}
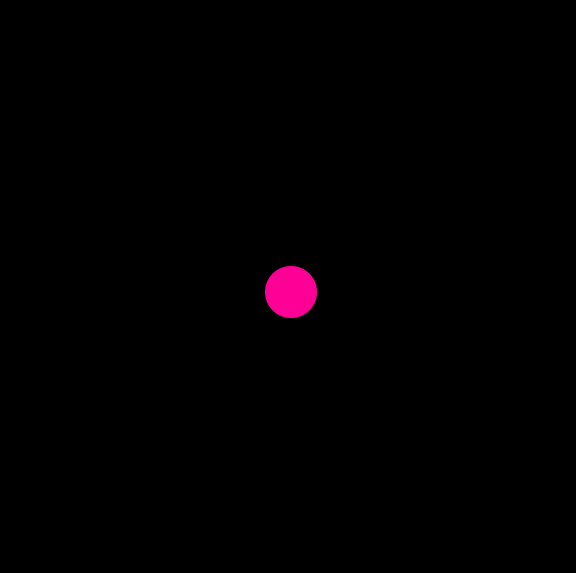
SIMPLE PAINT
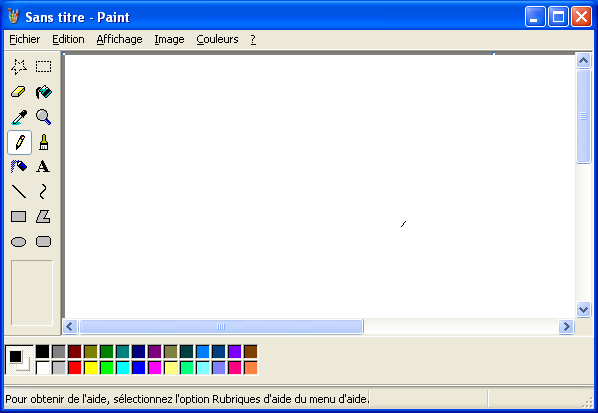
//Code to create a simple paint with
color couleur = color(255);
float epaisseur = 4;
int i=0;
void setup() {
size(700, 700);
background(0);
}
void draw() {
dessiner();
indicateur();
colorPicker();
gomme();
erase();
sizeStroke();
enregistrer();
}
void gomme() {
if (keyPressed == true) {
if (key == 'a'|| key == 'A' && mousePressed == true) {
stroke(0);
strokeWeight(20);
line(pmouseX, pmouseY, mouseX, mouseY);
}
}
}
void indicateur() {
noStroke();
fill(couleur);
rect(0, 0, 40, 40);
}
void dessiner() {
if (mousePressed == true) {
strokeWeight(epaisseur);
stroke(couleur);
line(pmouseX, pmouseY, mouseX, mouseY);
}
}
void colorPicker() {
if (mousePressed == true && mouseX <= 40 && mouseY <= 40) {
couleur=color(random(255), random(255), random(255));
}
}
void erase() {
if (keyPressed == true) {
if (key == 'E' || key == 'e') {
background(0);
}
}
}
void sizeStroke() {
if (keyPressed == true) {
if (key == CODED) {
if (keyCode == UP) {
epaisseur = epaisseur+0.1;
}
if (keyCode == DOWN) {
epaisseur = epaisseur-0.1;
if (epaisseur <= 0.5) epaisseur = 0.5;
}
}
}
}
void enregistrer() {
if (keyPressed == true) {
if (key == 'S' || key == 's') {
save(i+"dessin.png");
println("save");
i++;
}
}
}